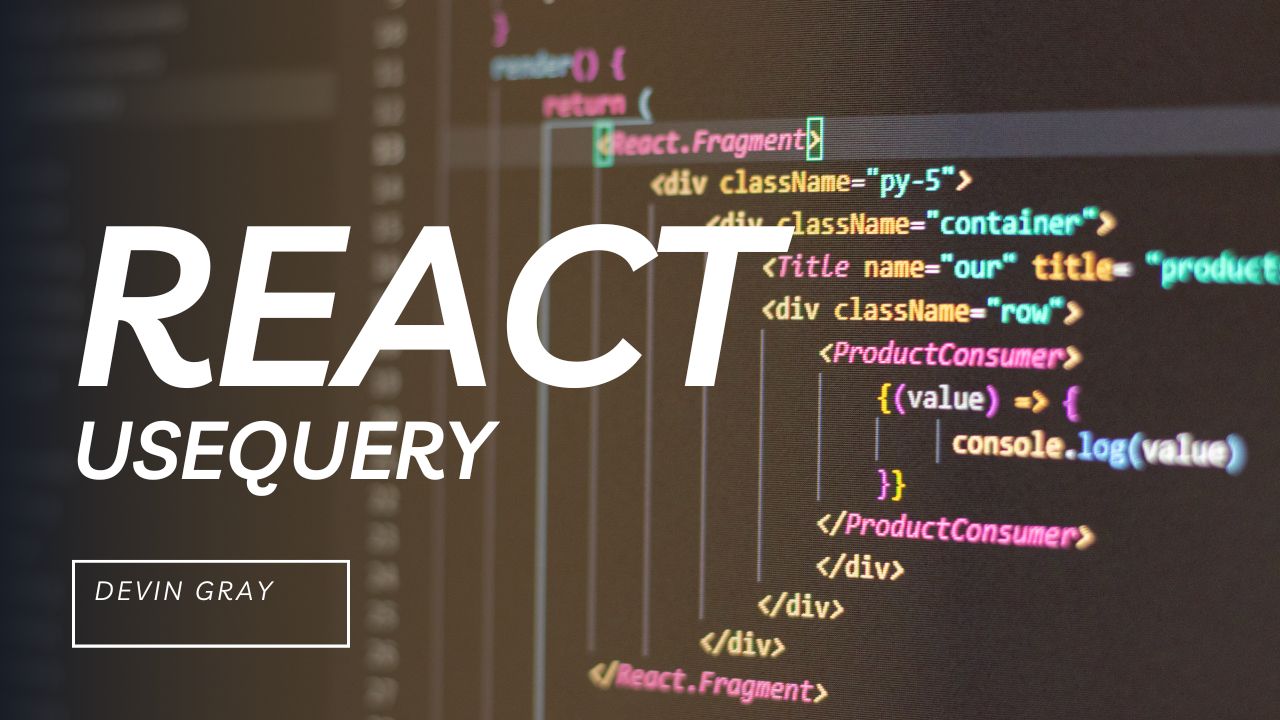
- 23 Sep, 2023
- 9 min read
Introduction to React Query’s useQuery Hook
React Query is a powerful library that provides a set of hooks and utilities to manage data fetching and state management in React applications. One of the most widely used hooks in React Query is the useQuery
hook, which allows developers to easily fetch and manage data from remote APIs.
In this blog post, we will explore the useQuery
hook in detail, covering its installation, setup, advantages, disadvantages, and various use cases with code examples.
Installation and Setup
To start using React Query and its useQuery
hook, we need to install the library first. We can do this using npm or yarn:
npm install react-query
# or
yarn add react-query
Once installed, we need to wrap our application with the QueryClientProvider
component provided by React Query. This component serves as a context provider for the library and should be placed at the top of the component tree. Here’s an example setup in an App
component:
import React from 'react';
import { QueryClient, QueryClientProvider } from 'react-query';
const queryClient = new QueryClient();
function App() {
return (
<QueryClientProvider client={queryClient}>
{/* Rest of the app */}
</QueryClientProvider>
);
}
export default App;
Advantages of using React Query
React Query provides several advantages when it comes to data fetching and state management:
- Automatic caching: React Query automatically caches fetched data and provides strategies for cache invalidation and data refetching. This greatly improves performance and reduces unnecessary network requests.
- Real-time updates: React Query integrates seamlessly with GraphQL and real-time APIs. It allows us to subscribe to server-side events and automatically update the UI when data changes.
- Mutation handling: React Query provides mutation hooks and utilities to handle data mutation and synchronization with the server. It simplifies the process of updating, creating, and deleting data.
- Pagination and infinite scrolling: React Query offers built-in support for pagination and infinite scrolling. It handles the logic of fetching and appending paginated data effortlessly, making it easy to implement these features in our application.
- Error handling and retries: React Query includes robust error handling and retry mechanisms out-of-the-box. It provides options to handle network errors, define retry strategies, and display error states in the UI.
- Devtools integration: React Query offers an excellent set of developer tools to inspect and debug queries, mutations, and cache states. This helps developers track and monitor data fetching and state changes.
Exploring the useQuery Hook
Now that we have React Query installed and set up, let’s dive into the useQuery
hook and its various use cases with code examples.
The useQuery
hook accepts two parameters: a unique key to identify the query and a function that fetches the data from the API. Here’s an example of fetching a list of users from a remote API:
import React from 'react';
import { useQuery } from 'react-query';
function Users() {
const { data, isLoading, isError } = useQuery('users', () =>
fetch('/api/users').then((res) => res.json())
);
if (isLoading) {
return <div>Loading...</div>;
}
if (isError) {
return <div>Error fetching users</div>;
}
return (
<div>
{data.map((user) => (
<div key={user.id}>{user.name}</div>
))}
</div>
);
}
In the example above, the useQuery
hook fetches data from the /api/users
endpoint. It returns a data
object containing the fetched data, along with isLoading
and isError
flags to indicate the loading and error states of the query.
Additional Features and Use Cases
Querying with Parameters
The useQuery
hook also allows us to pass parameters to the query function. This is useful when fetching data based on dynamic values. Here’s an example of fetching a specific user based on an ID:
import React from 'react';
import { useQuery } from 'react-query';
function User({ userId }) {
const { data, isLoading, isError } = useQuery(['user', userId], () =>
fetch(`/api/users/${userId}`).then((res) => res.json())
);
// Rest of the code
}
In this example, we pass an array as the query key, where the first element is a string to identify the query (‘user’) and the second element is the dynamic userId
prop.
Manual Query Refetching
React Query provides a refetch
function to manually trigger a query refetch. This is useful when we want to update the data without waiting for the automatic refetching. Here’s an example:
import React from 'react';
import { useQuery } from 'react-query';
function Users() {
const { data, isLoading, refetch } = useQuery('users', () =>
fetch('/api/users').then((res) => res.json())
);
// Rest of the code
return (
<button onClick={() => refetch()}>Refetch Users</button>
);
}
In this example, we call the refetch
function when the button is clicked, triggering a manual refetch of the users.
Conclusion
React Query’s useQuery
hook is a powerful tool for managing data-fetching in React applications. It provides automatic caching, real-time updates, mutation handling, pagination support, error handling, and debugging tools. With its simple API and comprehensive feature set, React Query simplifies data-fetching and state management, making it an excellent choice for data-intensive applications.
To learn more about React Query’s useQuery
hook and other hooks and utilities provided by the library, refer to the official React Query documentation: React Query Docs.
I hope this blog post has been informative and helpful in understanding the useQuery
hook in React Query. Happy querying!