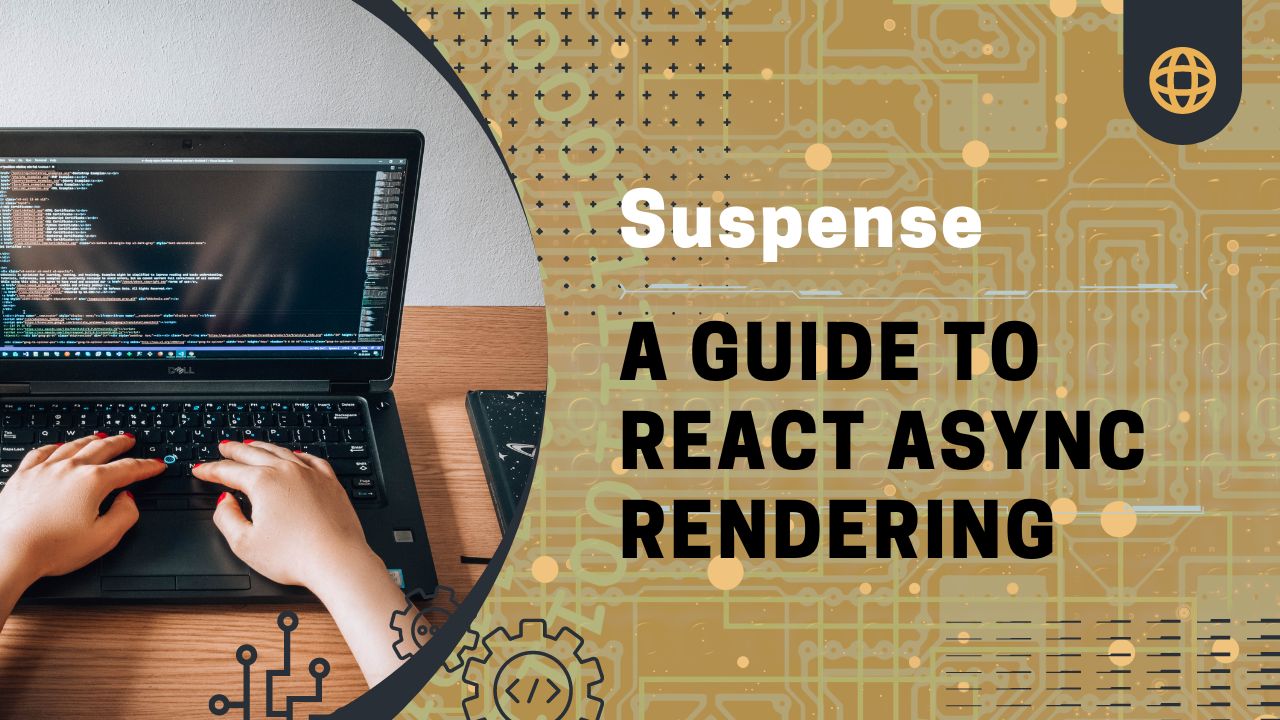
- 23 Sep, 2023
- read
Understanding React’s Suspense API: Empowering Smooth Asynchronous Rendering
Introduction
React’s Suspense API is a powerful feature that allows developers to handle async rendering in their React applications with ease. It improves the user experience by providing smooth transitions and fallback behaviors while components are being loaded. In this article, we will explore the key concepts and use cases of React’s Suspense API, demonstrating its potential with code examples.
What is React’s Suspense API? The Suspense API is a mechanism introduced in React 16.6 to simplify asynchronous rendering of components and manage their loading states. It allows React components to “suspend” rendering while they wait for data to load asynchronously. Suspense coordinates component loading and fallback rendering, ultimately rendering the component once the required data is available.
Key Concepts
To understand the Suspense API better, let’s explore its core concepts.
- Suspense: The Suspense component serves as a boundary for lazy-loaded components or resources. It ensures that the fallback UI is displayed while waiting for the required data or components to load asynchronously.
- Lazy Loading: Lazy loading refers to the technique of deferring the loading of a component until it is actually needed. It helps reduce the initial bundle size and improve performance by loading components only when necessary.
- Fallback UI: A fallback UI is a placeholder UI that is shown while the requested data or component is being fetched or loaded asynchronously. It gives users a visual feedback that something is happening and helps create a seamless experience.
Use Cases
Now, let’s explore some use cases where React’s Suspense API can be highly beneficial.
- Code-Splitting with Lazy Loading: Code-splitting is a technique to split your application’s code into smaller chunks that are loaded only when needed. By combining code-splitting with lazy loading, you can significantly improve the initial load time of your application.
Here’s an example using React’s Suspense API and the dynamic import() function to lazily load a component:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function MyComponent() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
In this example, the ‘LazyComponent’ is only loaded when ‘MyComponent’ is rendered. The ‘Suspense’ component ensures that the fallback UI (in this case, a simple “Loading…” message) is displayed until the ‘LazyComponent’ finishes loading.
- Data Fetching: React’s Suspense API can also be used for handling asynchronous data fetching. It allows you to fetch data in a declarative way and handle fallbacks while data is being loaded.
Here’s an example using React’s Suspense API and the ‘react-query’ library for data fetching:
const fetchUserData = async () => {
const response = await fetch('/api/user');
return response.json();
};
function UserComponent() {
const user = useQuery('user', fetchUserData);
return (
<div>
<Suspense fallback={<div>Loading user data...</div>}>
<UserInfo user={user.data} />
</Suspense>
</div>
);
}
In this example, the ‘useQuery’ hook from ‘react-query’ fetches the user data asynchronously. The ‘Suspense’ component ensures that the fallback UI (“Loading user data…”) is displayed until the ‘user’ data is available.
Conclusion
React’s Suspense API provides a convenient way to handle asynchronous rendering in React applications. With its ability to handle code-splitting, lazy loading, and data fetching, Suspense enables us to build more efficient and seamless user experiences. By using proper loading states, fallback UIs, and error boundaries, we can deliver smooth transitions and enhance overall performance.
Note: This blog post was written by Devin Gray, a Software Engineer. The code examples and concepts mentioned in this article are based on personal experience and knowledge acquired from the official React documentation.