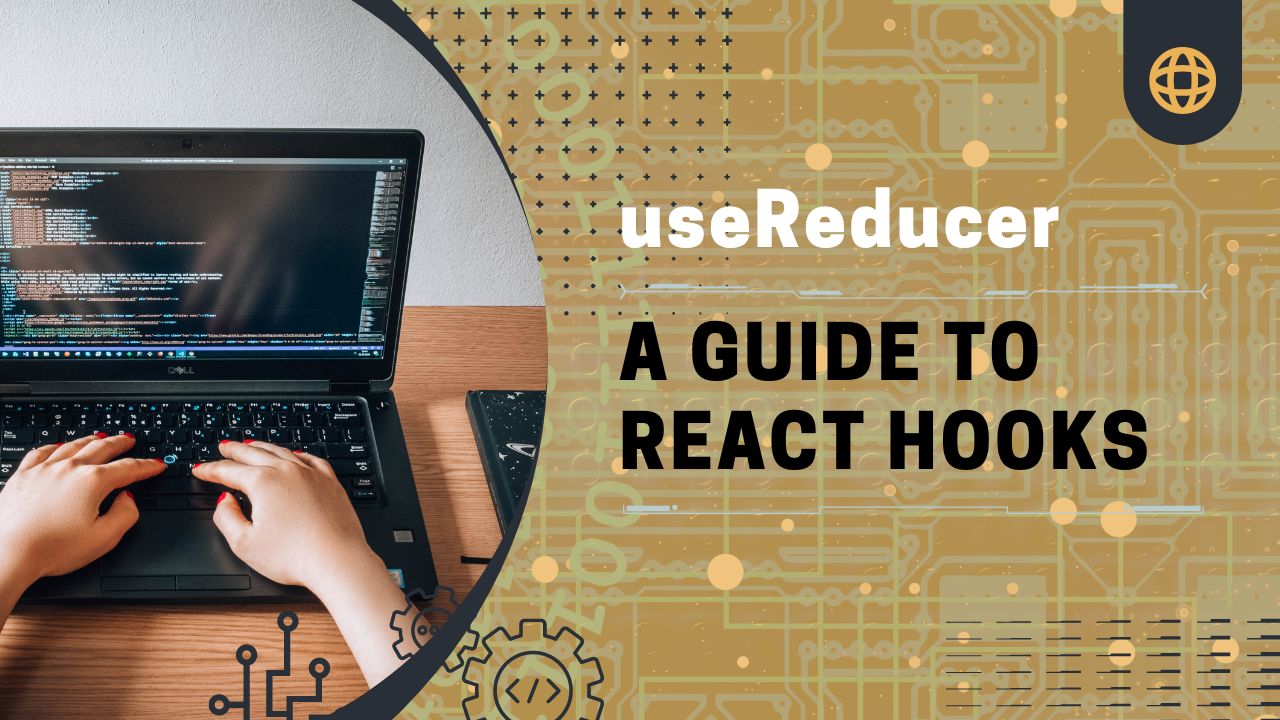
- 23 Sep, 2023
- 6 min read
Understanding React’s useReducer Hook
Introduction
React is a popular JavaScript library for building user interfaces, and it provides several built-in hooks that help manage state and logic within functional components. One such powerful hook is useReducer
, which allows us to handle complex state updates using a reducer function. In this article, we will explore the useReducer
hook, its use cases, and how to use it effectively in React applications.
What is a Reducer?
Before diving into useReducer
, let’s first understand what a reducer is. A reducer is a function that takes in the current state and an action as arguments and returns a new state. It follows the pattern:
(state, action) => newState
The idea behind a reducer is to have a predictable way of updating state based on the current state and the action being performed.
Understanding useReducer:
The useReducer
hook is a built-in React hook that is primarily used to manage state in complex scenarios. It is an alternative to the useState
hook and provides more control over state updates. Instead of updating the state directly, useReducer
takes in a reducer function and an initial state value.
Here is the syntax for using useReducer
:
const [state, dispatch] = useReducer(reducer, initialState);
Let’s break down the parameters:
reducer
: A function that takes in the current state and an action, and returns a new state.initialState
: The initial state value.
The useReducer
hook returns an array with two elements: state
and dispatch
.
state
: The current state value.dispatch
: A function used to dispatch actions to the reducer function.
Use Cases for useReducer
- Complex State Management:
The primary use case of
useReducer
is when you have complex state management logic that involves multiple related state variables. By using a reducer function, you can handle all state updates in one place, making your code more organized and easier to reason about. - Global State Management:
useReducer
is also suitable for managing global state in your application. By creating a global context and usinguseReducer
in combination with theuseContext
hook, you can easily share state across different components without the need for prop drilling. - Optimizing Performance:
In certain scenarios, where multiple state updates are happening in rapid succession, the
useState
hook may cause unnecessary re-renders. By usinguseReducer
, you can optimize performance by batching multiple state updates into one, resulting in a single re-render.
Example of useReducer
Let’s explore a simple example demonstrating the use of useReducer
.
import React, { useReducer } from 'react';
const initialState = 0;
function reducer(state, action) {
switch (action.type) {
case 'increment':
return state + 1;
case 'decrement':
return state - 1;
case 'reset':
return initialState;
default:
throw new Error('Invalid action type');
}
}
function Counter() {
const [count, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
<button onClick={() => dispatch({ type: 'reset' })}>Reset</button>
</div>
);
}
export default Counter;
In this example, we have a simple counter component that uses useReducer
to manage the state. The reducer function takes the current state and the action type, and based on the action type, it returns the updated state. The component renders the current count value and provides buttons to increment, decrement, and reset the count value.
Conclusion
React’s useReducer
hook is a powerful tool for managing complex state logic in functional components. By using a reducer function, you can handle state updates predictably and efficiently. It is particularly useful when dealing with complex state management, global state, or performance optimization. Understanding and utilizing useReducer
effectively will help you write cleaner, more maintainable React code.
References
- React Documentation: Using the State Hook
- React Documentation: Using the Effect Hook