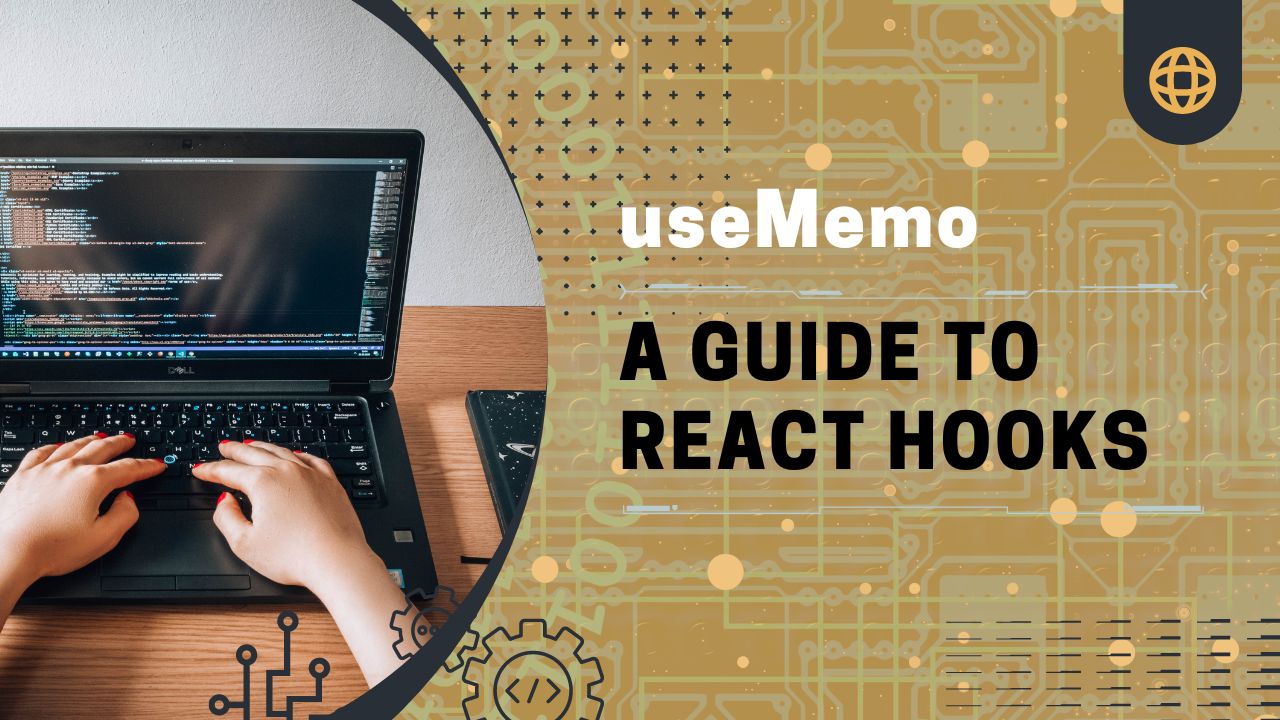
- 23 Sep, 2023
- read
Understanding React’s useMemo Hook – Optimizing Performance and Reducing Wasted Computations
Introduction:
As a software engineer, optimizing performance is often a critical task. In React applications, unnecessary computations can lead to performance issues, especially when dealing with heavy calculations or data manipulations. React’s useMemo hook provides a solution by memorizing the result of a function so that it is only recomputed when its dependencies change. In this blog post, we’ll explore the proper use cases for the useMemo hook and how it can help us optimize our React applications.
What is useMemo?
React’s useMemo hook allows us to memoize the result of a function by caching its return value. It takes two arguments: a function and an array of dependencies. Whenever any of the dependencies change, useMemo will recompute the function and return the cached result.
Proper use cases for useMemo:
- Computing derived data: Consider a scenario where we have an expensive data computation that is derived from other state or property values. By wrapping this calculation in useMemo, we can avoid recomputing the data unnecessarily.
import React, { useMemo } from 'react';
const MyComponent = ({ items }) => {
const expensiveComputation = useMemo(() => {
// Expensive calculation based on items
let result = 0;
for (let i = 0; i < items.length; i++) {
result += items[i] * 2;
}
return result;
}, [items]);
// Use expensiveComputation in the component
return <div>{expensiveComputation}</div>;
};
- Preventing unnecessary renders: In React, component re-renders can be triggered by changes in the component’s props or state. Using useMemo can prevent unnecessary render cycles by memoizing the result of a function. This is particularly useful in scenarios where the render output solely depends on specific props or state values.
import React, { useMemo } from 'react';
const MyComponent = ({ count }) => {
const renderOutput = useMemo(() => {
return <div>The count is: {count}</div>;
}, [count]);
// Use renderOutput in the component
return <div>{renderOutput}</div>;
};
- Optimizing intensive calculations: When dealing with heavy computations or complex algorithms, it is essential to optimize performance. useMemo can be used to improve the performance of such calculations by caching the result and avoiding unnecessary recomputations. This can greatly enhance the overall responsiveness of your application.
import React, { useMemo } from 'react';
const MyComponent = ({ data }) => {
const optimizedComputation = useMemo(() => {
// Perform intensive calculations based on data
let result = '';
// ...
return result;
}, [data]);
// Use optimizedComputation in the component
return <div>{optimizedComputation}</div>;
};
When NOT to Use a useMemo Hook
The useMemo
hook in React is used to memoize the result of a computation and prevent unnecessary re-computations. While useMemo
can be useful in certain scenarios, there are cases when it may not be necessary or recommended:
- Simple calculations: If the computation you need to perform is very simple and lightweight, the performance impact of re-calculating it on each render might be negligible. In such cases, it’s better to not use
useMemo
and keep the code simpler. - Render-only logic: If the computation is related to rendering logic only and doesn’t have any side effects or dependencies on other variables or props, then you don’t need to use
useMemo
. React’s rendering process is already optimized to efficiently render components, so directly incorporating the computation in the render function should be sufficient. - No expensive operations: If the computation you want to memoize is not expensive in terms of time complexity or resource usage, using
useMemo
may not provide any noticeable performance improvement. It’s usually best to focus on optimizing the parts of your codebase that have a significant impact on performance. - Static values: When the value you are computing doesn’t change over time and remains static, there is no need to use
useMemo
. Instead, you can directly assign the computed value to a variable or prop within the component.
Remember, the decision to use or not use useMemo
depends on the specific use case and performance considerations. It’s always a good practice to profile and benchmark your application to determine the impact of using useMemo
in different scenarios.
Conclusion:
React’s useMemo hook is a powerful tool for optimizing performance in React applications. By memoizing the result of a function, we can avoid unnecessary recomputations and prevent needless render cycles. This is particularly useful when dealing with expensive data computations, preventing unnecessary renders, and optimizing performance-intensive calculations. Proper use of useMemo can lead to improved responsiveness and a smoother user experience.
Remember to use useMemo when you have a function that is computationally expensive, and its return value depends on specific dependencies that change over time. By utilizing useMemo effectively, you can significantly enhance the performance of your React applications.
Further Resources: