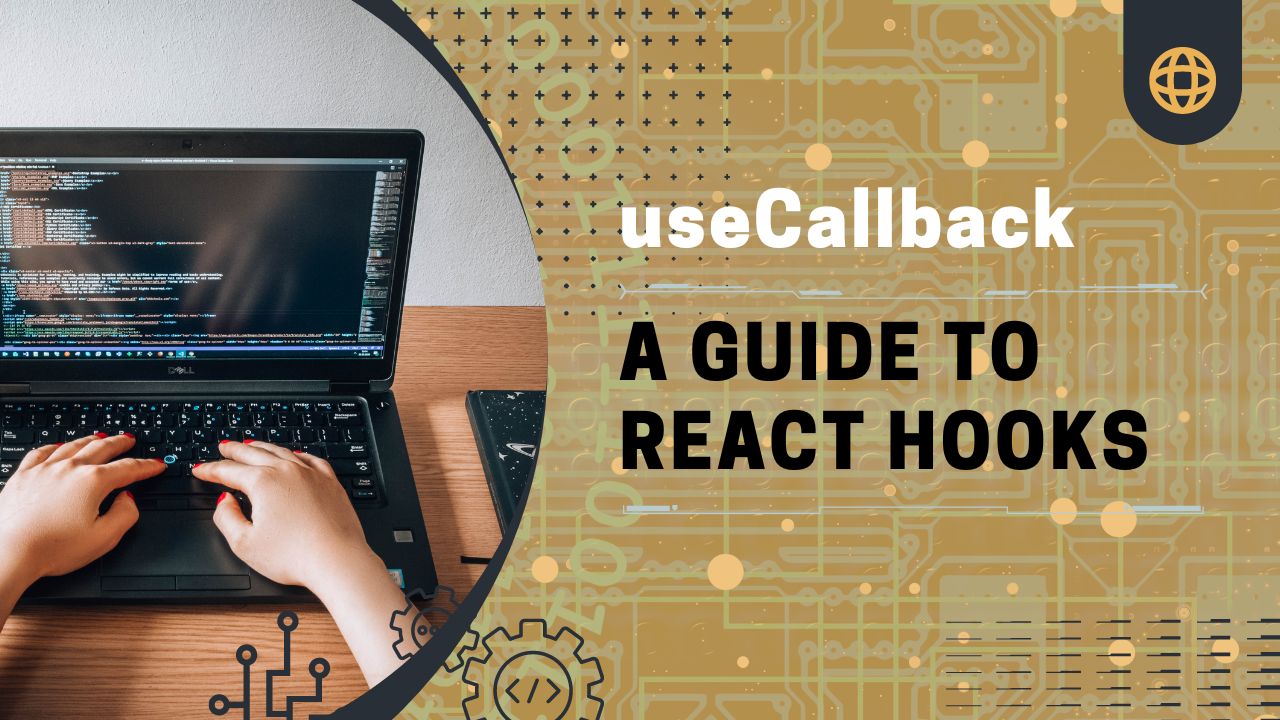
- 23 Sep, 2023
- read
React’s useCallback Hook: Improving Performance and Preventing Unnecessary Rerenders
React’s useCallback
hook is a powerful tool that can greatly improve the performance of your React applications. It is especially useful when working with components that rely on callbacks, such as event handlers. In this article, we’ll explore what useCallback
does, how it works, and the proper use cases for this hook.
Understanding Callback Functions
Before we dive into the useCallback
hook, it’s important to understand what callback functions are and why they are commonly used in React components. In JavaScript, a callback function is a function that is passed as an argument to another function and is invoked at a later point in time. Callback functions are often used to handle asynchronous events or to perform actions based on user interactions, such as button clicks.
In React, we often use callback functions to update the state of a component or to pass data between parent and child components. However, if these callback functions are created within the component’s body, they are redefined during every render. This can lead to unnecessary rerenders of child components and negatively impact performance.
Introducing useCallback
To prevent unnecessary rerenders and improve performance, React provides the useCallback
hook. useCallback
is used to memoize a callback function so that it is only recreated when its dependencies change. By doing so, we can ensure that the same instance of the callback function is passed to child components, avoiding unnecessary rerenders caused by callback function changes.
Here is an example to illustrate how useCallback
can be used:
import React, { useCallback } from "react";
function MyComponent() {
const handleClick = useCallback(() => {
// Handle click event
}, []);
return <button onClick={handleClick}>Click me</button>;
}
In the example above, handleClick
is the callback function that is memoized using useCallback
. The second argument of useCallback
is an array of dependencies. If any of these dependencies change, the callback function will be recreated. By providing an empty array as the second argument, we effectively memoize the callback function so that it is not recreated during subsequent renders.
When to Use useCallback
Now that we understand how useCallback
works, let’s explore some common use cases for this hook.
1. Memoizing Callback Functions
As mentioned earlier, useCallback
is primarily used to memoize callback functions. This is useful when passing callbacks down to child components, as it ensures that child components only re-render when necessary.
import React, { useCallback } from "react";
function ParentComponent() {
const handleClick = useCallback(() => {
// Handle click event
}, []);
return <ChildComponent onClick={handleClick} />;
}
function ChildComponent({ onClick }) {
// Render child component
}
In this example, the handleClick
function is memoized using useCallback
in the ParentComponent
. When ParentComponent
re-renders, the same instance of handleClick
is passed down to ChildComponent
. If handleClick
was not memoized, ChildComponent
would be unnecessarily re-rendered whenever ParentComponent
re-renders.
2. Optimizing Complex Computations
useCallback
can also be used to optimize complex computations that are performed within a callback. Consider the following example:
import React, { useCallback, useState } from "react";
function MyComponent() {
const [count, setCount] = useState(0);
const calculateResult = useCallback(() => {
// Perform complex computation based on count
return count * 2;
}, [count]);
return (
<div>
<p>Count: {count}</p>
<p>Result: {calculateResult()}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In this example, the calculateResult
function depends on the count
state. By passing count
as a dependency to useCallback
, calculateResult
will only be recreated when count
changes. This prevents unnecessary recomputation of the result when other state variables or props change.
3. Avoiding Infinite Loops
Another important use case for useCallback
is to avoid infinite loops caused by dependencies that change too frequently. When creating a callback function that itself depends on other state variables or props, useCallback
can prevent infinite loops.
import React, { useState, useCallback } from "react";
function MyComponent() {
const [value, setValue] = useState("");
const handleChange = useCallback((event) => {
setValue(event.target.value);
}, []);
return <input type="text" value={value} onChange={handleChange} />;
}
In this example, the handleChange
function is memoized using useCallback
. If we omitted the useCallback
hook, handleChange
would be redefined on every render, causing the input
element to lose focus whenever the user types. By memoizing handleChange
using useCallback
, we ensure that the same instance of the function is used, preventing unnecessary rerenders and maintaining focus within the input
element.
When NOT to Use A useCallback Hook
The useCallback
hook in React is used to memoize a function so that it is only re-created when one of its dependencies changes. It can be useful to improve performance and prevent unnecessary re-renders in certain cases. However, there are situations when using useCallback
may not be necessary or even recommended:
- Functions that don’t have dependencies: If a function doesn’t rely on any variables or props from the component, there is no need to use
useCallback
to memoize it. In such cases, it’s better to define the function outside the component so that it’s only created once. - Performance overhead: Memoizing functions using
useCallback
does introduce some performance overhead, as it requires creating and maintaining a memoization cache. If the function is not called very frequently or if the performance impact is negligible, it might be better to avoid usinguseCallback
to keep the code simpler. - Functions needed in child components: If a function is only used internally within a component and is not passed down as a prop to child components, there is no need to memoize it with
useCallback
. The child components won’t be affected by changes in the parent component’s internal function. - Functions that depend on all or most props: If a function relies on all or most of the props passed to a component, memoizing it with
useCallback
may not provide significant benefits. In such cases, the function will be re-created whenever any prop changes, making the memoization less effective.
Remember, the decision to use or not use useCallback
depends on the specific use case and performance considerations. It’s always a good practice to profile and benchmark your application to determine the impact of using useCallback
in different scenarios.
Conclusion
React’s useCallback
hook is a powerful tool that can greatly improve the performance of your React applications. By memoizing callback functions, useCallback
prevents unnecessary rerenders of child components and optimizes complex computations. Additionally, useCallback
helps avoid infinite loops caused by dependencies that change too frequently.
In summary, here are the key takeaways:
- Use
useCallback
to memoize callback functions and prevent unnecessary rerenders. - Provide the dependencies of the callback function as the second argument to
useCallback
. - Memoize computationally expensive functions to optimize performance.
- Prevent infinite loops caused by changing dependencies using
useCallback
.
With useCallback
, you can ensure that your React components are efficient, performant, and responsive.
References: